Extra material: Optimal Growth Portfolios with Risk Aversion#
Among the reasons why Kelly was neglected by investors were high profile critiques by the most famous economist of the 20th Century, Paul Samuelson. Samuelson objected on several grounds, among them is a lack of risk aversion that results in large bets and risky short term behavior, and that Kellyâs result is applicable to only one of many utility functions that describe investor preferences. The controversy didnât end there, however, as other academic economists, including Harry Markowitz, and practitioners found ways to adapt the Kelly criterion to investment funds.
This notebook presents solutions to Kellyâs problem for optimal growth portfolios using exponential cones. A significant feature of this notebook is the inclusion of a risk constraint recently proposed by Boyd and coworkers. These notes are based on recent papers such as Cajas (2021), Busseti, Ryu and Boyd (2016), Fu, Narasimhan, and Boyd (2017). Additional bibliographic notes are provided at the end of the notebook.
# install dependencies and select solver
%pip install -q amplpy numpy pandas
SOLVER_CONIC = "mosek" # ipopt, mosek, knitro
from amplpy import AMPL, ampl_notebook
ampl = ampl_notebook(
modules=["coin", "mosek"], # modules to install
license_uuid="default", # license to use
) # instantiate AMPL object and register notebook magic
Financial Data#
We begin by reading historical prices for a selected set of trading symbols using yfinance.
While it would be interesting to include an international selection of financial indices and assets, differences in trading and bank holidays would involve more elaborate coding. For that reason, the following cell has been restricted to indices and assets trading in U.S. markets.
# run this cell to install yfinance
%pip install yfinance --upgrade -q
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import datetime
import yfinance as yf
# symbols as used by Yahoo Finance
symbols = {
# selected indices
"^GSPC": "S&P 500",
"^IXIC": "Nasdaq",
"^DJI": "Dow Jones Industrial",
"^RUT": "Russell 2000",
# selected stocks
"AXP": "American Express",
"AMGN": "Amgen",
"AAPL": "Apple",
"BA": "Boeing",
"CAT": "Caterpillar",
"CVX": "Chevron",
"JPM": "JPMorgan Chase",
"MCD": "McDonald's",
"MMM": "3 M",
"MSFT": "Microsoft",
"PG": "Proctor & Gamble",
"XOM": "ExxonMobil",
}
# years of testing and training data
n_test = 1
n_train = 2
# get today's date
today = datetime.datetime.today().date()
# training data dates
end = today - datetime.timedelta(int(n_test * 365))
start = end - datetime.timedelta(int((n_test + n_train) * 365))
# get training data
S = yf.download(list(symbols.keys()), start=start, end=end)["Adj Close"]
# compute gross returns
R = S / S.shift(1)
R.dropna(inplace=True)
[*********************100%***********************] 16 of 16 completed
# plot
fig, ax = plt.subplots(2, 1, figsize=(8, 6), sharex=True)
S.divide(S.iloc[0] / 100).plot(ax=ax[0], grid=True, title="Normalized Prices")
ax[0].legend(loc="center left", bbox_to_anchor=(1.0, 0.5), prop={"size": 8})
R.plot(ax=ax[1], grid=True, title="Gross Returns", alpha=0.5).legend([])
fig.tight_layout()
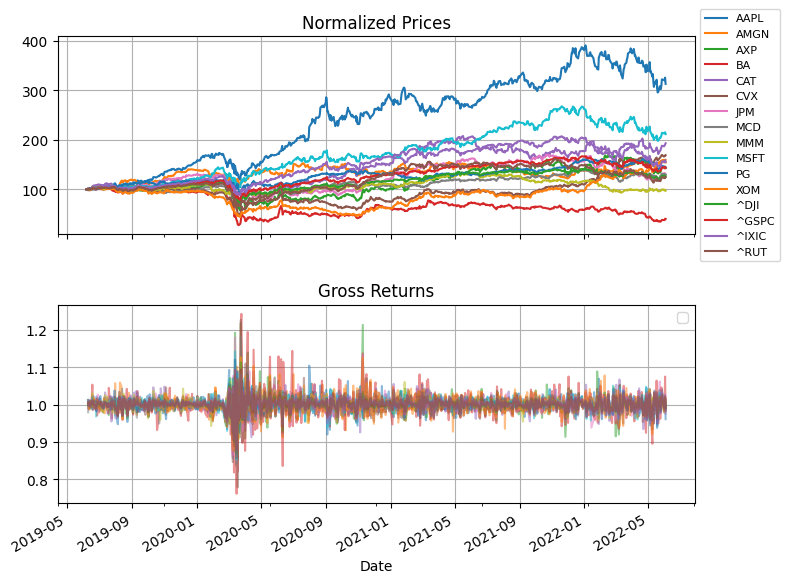
Portfolio Design for Optimal Growth#
Model#
Here we are examining a set \(N\) of financial assets trading in efficient markets. The historical record consists of a matrix \(R \in \mathbb{R}^{T\times N}\) of gross returns where \(T\) is the number of observations.
The weights \(w_n \geq 0\) for \(n\in N\) denote the fraction of the portfolio invested in asset \(n\). Any portion of the portfolio not invested in traded assets is assumed to have a gross risk-free return \(R_f = 1 + r_f\), where \(r_f\) is the return on a risk-free asset.
Assuming the gross returns are independent and identically distributed random variables, and the historical data set is representative of future returns, the investment model becomes
Note this formulation allows the sum of weights \(\sum_{n\in N} w_n\) to be greater than one. In that case the investor would be investing more than the value of the portfolio in traded assets. In other words the investor would be creating a leveraged portfolio by borrowing money at a rate \(R_f\). To incorporate a constraint on the degree of leveraging, we introduce a constraint
where \(E_M\) is the âequity multiplier.â A value \(E_M \leq 1\) restricts the total investment to be less than or equal to the equity available to the investor. A value \(E_M > 1\) allows the investor to leverage the available equity by borrowing money at a gross rate \(R_f = 1 + r_f\).
Using techniques demonstrated in other examples, this model can be reformulated with exponential cones.
For the risk constrained case, we consider a constraint
where \(\lambda\) is a risk aversion parameter. Assuming the historical returns are equiprobable
The risk constraint is satisfied for any \(w_n\) if the risk aversion parameter \(\lambda=0\). For any value \(\lambda > 0\) the risk constraint has a feasible solution \(w_n=0\) for all \(n \in N\). Recasting as a sum of exponentials,
Using the \(q_t \leq \log(R_t)\) as used in the examples above, and \(u_t \geq e^{- \lambda q_t}\), we get the risk constrained model optimal log growth.
Given a risk-free rate of return \(R_f\), a maximum equity multiplier \(E_M\), and value \(\lambda \geq 0\) for the risk aversion, risk constrained Kelly portfolio is given the solution to
The following cells demonstrate an AMPL implementation of the model with the Mosek solver.
AMPL Implementation#
The AMPL implementation for the risk-constrained Kelly portfolio accepts three parameters, the risk-free gross returns \(R_f\), the maximum equity multiplier, and the risk-aversion parameter.
%%writefile kelly_portfolio.mod
param Rf;
param EM;
param lambd;
# index lists
set T;
set N;
param Rloc{T, N};
# decision variables
var q{T};
var w{N} >= 0;
# objective
maximize ElogR:
sum {t in T} q[t] / card(T);
# conic constraints on return
var R{t in T}
= Rf + sum {n in N} w[n]*(Rloc[t, n] - Rf);
s.t. C{t in T}:
R[t] >= exp(q[t]);
# risk constraints
var u{T};
s.t. USum:
sum {t in T} u[t] / card(T) <= Rf**(-lambd);
s.t. RT{t in T}:
u[t] >= exp( -lambd*q[t] );
# equity multiplier constraint
s.t. WSum:
sum {n in N} w[n] <= EM;
Overwriting kelly_portfolio.mod
def kelly_portfolio(R, Rf=1, EM=1, lambd=0):
ampl = AMPL()
ampl.read("kelly_portfolio.mod")
ampl.param["Rf"] = Rf
ampl.param["EM"] = EM
ampl.param["lambd"] = lambd
# index lists
ampl.set["T"] = [str(t) for t in R.index]
ampl.set["N"] = [str(n) for n in R.columns]
ampl.param["Rloc"] = {
(str(t), str(n)): R.at[t, n]
for i, t in enumerate(R.index)
for j, n in enumerate(R.columns)
}
ampl.option["solver"] = SOLVER_CONIC
ampl.solve()
return ampl
def kelly_report(ampl):
# print report
s = f"""
Risk Free Return = {100*(np.exp(252*np.log(ampl.get_value('Rf'))) - 1):0.2f}
Equity Multiplier Limit = {ampl.get_value('EM'):0.5f}
Risk Aversion = {ampl.get_value('lambd'):0.5f}
Portfolio
"""
w = ampl.get_variable("w").to_dict()
Rvar = ampl.get_variable("R").to_dict()
s += "\n".join([f"{n:8s} {symbols[n]:30s} {100*w[n]:8.2f} %" for n in w.keys()])
s += f"""
{'':8s} {'Risk Free':30s} {100*(1 - sum(w[n] for n in w.keys())):8.2f} %
Annualized return = {100*(np.exp(252*ampl.get_value('ElogR')) - 1):0.2f} %
"""
print(s)
df = pd.DataFrame(
pd.Series([Rvar[str(t)] for t in R.index]), columns=["Kelly Portfolio"]
)
df.index = R.index ## original index are the DateTimeStamps
fix, ax = plt.subplots(1, 1, figsize=(8, 8))
S.divide(S.iloc[0] / 100).plot(
ax=ax,
logy=True,
grid=True,
title="Normalized Prices",
alpha=0.6,
lw=0.4,
ls="--",
)
df.cumprod().multiply(100).plot(ax=ax, lw=3, grid=True)
ax.legend(
[symbols[n] for n in R.columns] + ["Kelly Portfolio"],
bbox_to_anchor=(1.05, 1.05),
)
d = S.index[-1]
print(d)
for n in w.keys():
y = 100 * S[n].iloc[-1] / S[n].iloc[0]
print(n, 100 * S[n].iloc[-1] / S[n].iloc[0])
ax.text(d, y, n)
# parameter values
Rf = np.exp(np.log(1.0) / 252)
EM = 1
lambd = 10
m = kelly_portfolio(R, Rf, EM, lambd)
kelly_report(m)
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115058867
0 simplex iterations
22 barrier iterations
Risk Free Return = 0.00
Equity Multiplier Limit = 1.00000
Risk Aversion = 10.00000
Portfolio
AAPL Apple 57.78 %
AMGN Amgen 0.00 %
AXP American Express 0.00 %
BA Boeing 0.00 %
CAT Caterpillar 18.97 %
CVX Chevron 0.00 %
JPM JPMorgan Chase 0.00 %
MCD McDonald's 0.00 %
MMM 3 M 0.00 %
MSFT Microsoft 0.00 %
PG Proctor & Gamble 0.00 %
XOM ExxonMobil 0.00 %
^DJI Dow Jones Industrial 0.00 %
^GSPC S&P 500 0.00 %
^IXIC Nasdaq 0.00 %
^RUT Russell 2000 0.00 %
Risk Free 23.25 %
Annualized return = 32.44 %
2022-06-03 00:00:00
AAPL 313.3368230987512
AMGN 154.57373693975774
AXP 143.59720714356118
BA 40.08344979462141
CAT 193.1338141274621
CVX 168.98770245792917
JPM 130.31933675829177
MCD 129.6343379131071
MMM 97.33192901788738
MSFT 211.75261984245606
PG 144.29053167324705
XOM 159.39669011089973
^DJI 126.61551678143734
^GSPC 142.9882963168018
^IXIC 155.1611360900202
^RUT 124.34379721298355
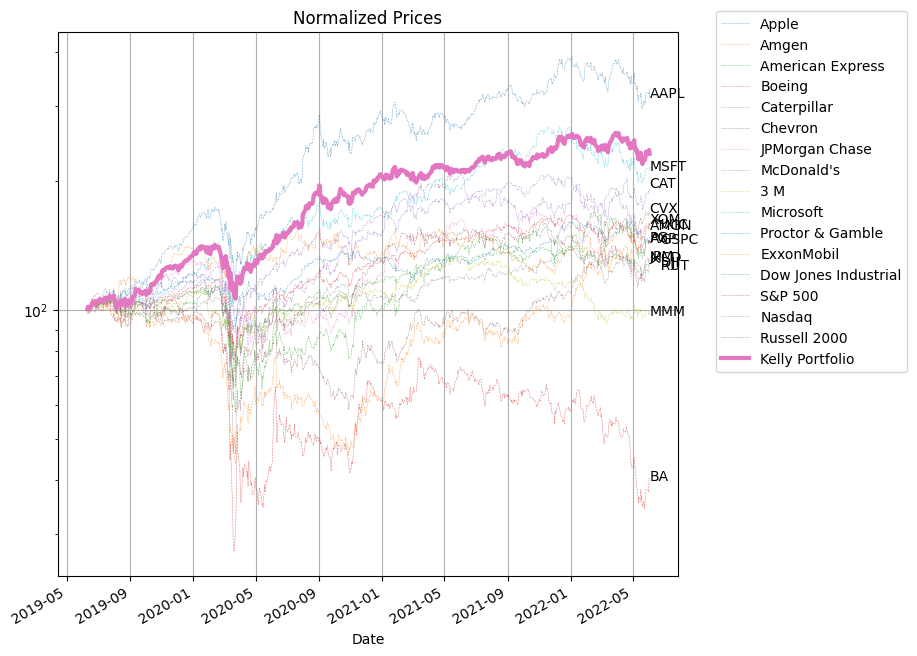
S.head()
AAPL | AMGN | AXP | BA | CAT | CVX | JPM | MCD | MMM | MSFT | PG | XOM | ^DJI | ^GSPC | ^IXIC | ^RUT | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Date | ||||||||||||||||
2019-06-07 | 46.121952 | 155.557022 | 114.483406 | 347.400238 | 112.699974 | 101.451523 | 96.646111 | 187.392517 | 142.644150 | 126.295914 | 98.509666 | 60.087990 | 25983.939453 | 2873.340088 | 7742.100098 | 1514.390015 |
2019-06-10 | 46.711361 | 155.619110 | 115.948608 | 347.498413 | 113.859032 | 102.127975 | 97.690834 | 183.580475 | 144.082504 | 127.449310 | 98.464378 | 60.353874 | 26062.679688 | 2886.729980 | 7823.169922 | 1523.560059 |
2019-06-11 | 47.252258 | 154.768219 | 116.487427 | 343.108063 | 115.253525 | 101.192619 | 97.991859 | 185.367935 | 144.613327 | 126.968742 | 99.062119 | 60.297466 | 26048.509766 | 2885.719971 | 7822.569824 | 1519.109985 |
2019-06-12 | 47.101887 | 155.636841 | 115.353081 | 340.849030 | 115.090538 | 100.374207 | 96.743507 | 186.890930 | 144.698929 | 126.382423 | 99.333824 | 59.644863 | 26004.830078 | 2879.840088 | 7792.720215 | 1519.790039 |
2019-06-13 | 47.092175 | 156.620621 | 115.192368 | 342.646423 | 115.153931 | 100.975510 | 96.982559 | 186.489700 | 144.561996 | 127.180168 | 100.447807 | 60.168556 | 26106.769531 | 2891.639893 | 7837.129883 | 1535.800049 |
Effects of the Risk-Aversion Parameter#
lambd = 10 ** np.linspace(0, 3)
results = [kelly_portfolio(R, Rf=1, EM=1, lambd=_) for _ in lambd]
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514729021
0 simplex iterations
12 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514730217
0 simplex iterations
11 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514731433
0 simplex iterations
12 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514732444
0 simplex iterations
12 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514732116
0 simplex iterations
13 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514731363
0 simplex iterations
13 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514730112
0 simplex iterations
13 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514731633
0 simplex iterations
13 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514732135
0 simplex iterations
14 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514730977
0 simplex iterations
14 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.00151473216
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514731721
0 simplex iterations
18 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001514732466
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001510090893
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001437622267
0 simplex iterations
27 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001295714115
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001158395522
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001032061346
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.000916678938
0 simplex iterations
36 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0008119779914
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0007174855375
0 simplex iterations
29 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0006326317078
0 simplex iterations
57 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0005567612961
0 simplex iterations
68 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.000489173801
0 simplex iterations
46 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0004291643027
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0003760314216
0 simplex iterations
30 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0003291057879
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0002877495441
0 simplex iterations
49 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0002513785857
0 simplex iterations
46 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0002194350789
0 simplex iterations
43 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0001914243691
0 simplex iterations
39 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0001668951972
0 simplex iterations
104 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0001454355608
0 simplex iterations
34 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0001266777712
0 simplex iterations
61 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.0001102993894
0 simplex iterations
50 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 9.601835593e-05
0 simplex iterations
51 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 8.353869664e-05
0 simplex iterations
54 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 7.267431243e-05
0 simplex iterations
120 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 6.320673287e-05
0 simplex iterations
74 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 5.496267527e-05
0 simplex iterations
81 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 4.780118333e-05
0 simplex iterations
121 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 4.15554229e-05
0 simplex iterations
95 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 3.612044892e-05
0 simplex iterations
119 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 3.137887756e-05
0 simplex iterations
244 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 2.726071662e-05
0 simplex iterations
197 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 2.368800934e-05
0 simplex iterations
294 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 2.059797531e-05
0 simplex iterations
168 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 1.789750665e-05
0 simplex iterations
123 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 1.555107149e-05
0 simplex iterations
242 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 1.349274934e-05
0 simplex iterations
200 barrier iterations
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(2, 1, figsize=(8, 4), sharex=True)
ax[0].semilogx(
[m.get_value("lambd") for m in results],
[100 * (np.exp(252 * m.get_value("ElogR")) - 1) for m in results],
)
ax[0].set_title("Portfolio Return vs Risk Aversion")
ax[0].set_ylabel("annual %")
ax[0].grid(True)
ax[1].semilogx(
[m.get_value("lambd") for m in results],
[
[w[str(n)] for n in R.columns]
for m in results
for w in [m.get_variable("w").to_dict()]
],
)
ax[1].set_ylabel("weights")
ax[1].set_xlabel("risk aversion $\lambda$")
ax[1].legend([symbols[n] for n in R.columns], bbox_to_anchor=(1.05, 1.05))
ax[1].grid(True)
ax[1].set_ylim(0, EM)
fig.tight_layout()
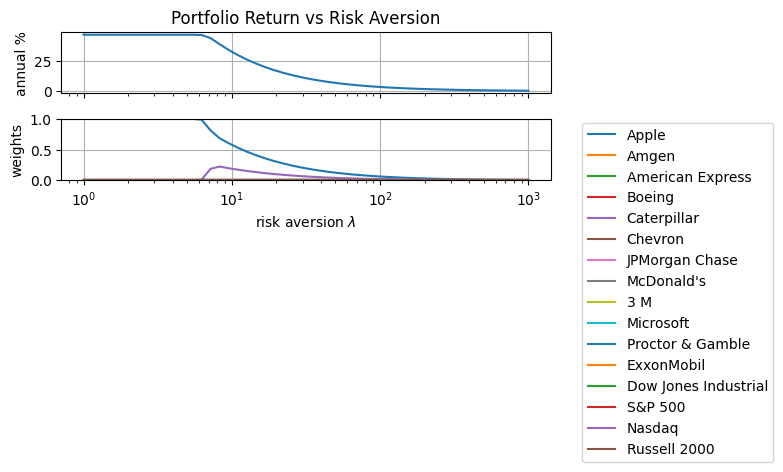
Effects of the Equity Multiplier Parameter#
EM = np.linspace(0.0, 2.0)
results = [kelly_portfolio(R, Rf=1, EM=_, lambd=10) for _ in EM]
MOSEK 10.0.43: MOSEK 10.0.43: unknown (0, problem status: 0), max number of iterations reached
0 simplex iterations
400 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 7.127764616e-05
0 simplex iterations
15 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0001417540358
0 simplex iterations
13 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0002114242562
0 simplex iterations
12 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0002802885279
0 simplex iterations
12 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0003483468736
0 simplex iterations
12 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0004156018916
0 simplex iterations
13 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0004820568272
0 simplex iterations
14 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0005477046862
0 simplex iterations
14 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0006125490061
0 simplex iterations
15 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0006765882375
0 simplex iterations
15 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.000739824828
0 simplex iterations
18 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0008022547016
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0008638806781
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0009247042986
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0009847215819
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001043934055
0 simplex iterations
25 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001078172194
0 simplex iterations
29 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001104816542
0 simplex iterations
27 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.00111506199
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115066018
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.00111506275
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115058768
0 simplex iterations
27 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115062686
0 simplex iterations
25 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115058794
0 simplex iterations
60 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115064775
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115059022
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115061998
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115064832
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115063757
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115062221
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115062519
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115063848
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115063227
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115061374
0 simplex iterations
30 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115059603
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115060952
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115058514
0 simplex iterations
39 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115060287
0 simplex iterations
18 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.0011150608
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115060207
0 simplex iterations
33 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115064884
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115064279
0 simplex iterations
31 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115061648
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115062476
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115058494
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115061564
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115063624
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115060886
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115060828
0 simplex iterations
21 barrier iterations
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(2, 1, figsize=(8, 4), sharex=True)
ax[0].plot(
[m.get_value("EM") for m in results],
[100 * (np.exp(252 * m.get_value("ElogR")) - 1) for m in results],
)
ax[0].set_title("Portfolio Return vs Equity Multiplier")
ax[0].set_ylabel("annual return %")
ax[0].grid(True)
ax[1].plot(
[m.get_value("EM") for m in results],
[
[w[str(n)] for n in R.columns]
for m in results
for w in [m.get_variable("w").to_dict()]
],
)
ax[1].set_ylabel("weights")
ax[1].set_xlabel("Equity Multiplier")
ax[1].legend([symbols[n] for n in R.columns], bbox_to_anchor=(1.05, 1.05))
ax[1].grid(True)
ax[1].set_ylim(
0,
)
fig.tight_layout()
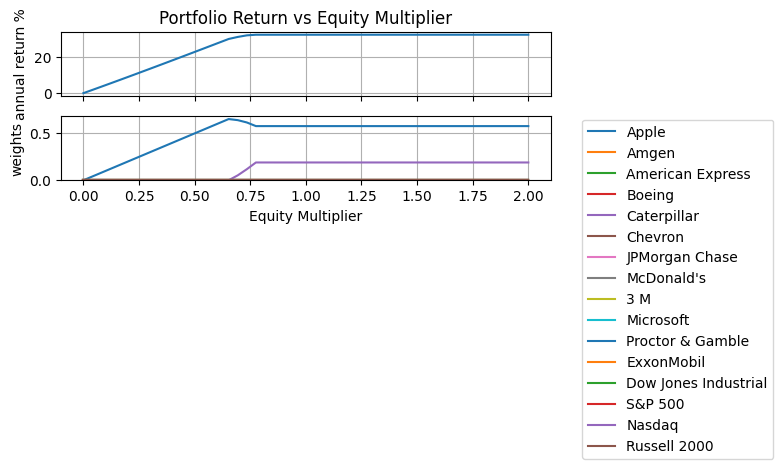
Effect of Risk-free Interest Rate#
Rf = np.exp(np.log(1 + np.linspace(0, 0.20)) / 252)
results = [kelly_portfolio(R, Rf=_, EM=1, lambd=10) for _ in Rf]
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001115058867
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001109229733
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001103644806
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001098315886
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001093231488
0 simplex iterations
25 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001088400657
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001083803179
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001079457309
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001075345323
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001071468947
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001067825963
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001064420867
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001061247108
0 simplex iterations
19 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001058296152
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001055577603
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001053077952
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001050797566
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001048747589
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001046908631
0 simplex iterations
20 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001045285188
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001043880982
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001042686638
0 simplex iterations
21 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001041703608
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001040928355
0 simplex iterations
45 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001040359883
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001040000051
0 simplex iterations
26 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.00103984141
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001039879451
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001040122591
0 simplex iterations
26 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001040556867
0 simplex iterations
45 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001041194597
0 simplex iterations
27 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001042019612
0 simplex iterations
29 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001043041453
0 simplex iterations
28 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001044258079
0 simplex iterations
33 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001045652626
0 simplex iterations
29 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001047237922
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001049012414
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001050967859
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001053110487
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001055426877
0 simplex iterations
24 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001057925246
0 simplex iterations
23 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal, stalling; objective 0.00106060001
0 simplex iterations
30 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001063452812
0 simplex iterations
39 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001066479571
0 simplex iterations
40 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001069678622
0 simplex iterations
32 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001073048927
0 simplex iterations
27 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001076589141
0 simplex iterations
26 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001080298143
0 simplex iterations
25 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001084143277
0 simplex iterations
22 barrier iterations
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 0.001088103524
0 simplex iterations
39 barrier iterations
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(2, 1, figsize=(8, 4), sharex=True)
Rf = np.exp(252 * np.log(np.array([_.get_value("Rf") for _ in results])))
ax[0].plot(Rf, [100 * (np.exp(252 * m.get_value("ElogR")) - 1) for m in results])
ax[0].set_title("Portfolio Return vs Risk-free Rate")
ax[0].set_ylabel("annual return %")
ax[0].grid(True)
ax[1].plot(
Rf,
[
[w[str(n)] for n in R.columns]
for m in results
for w in [m.get_variable("w").to_dict()]
],
)
ax[1].set_ylabel("weights")
ax[1].set_xlabel("Risk-free Rate")
ax[1].legend([symbols[n] for n in R.columns], bbox_to_anchor=(1.05, 1.05))
ax[1].grid(True)
ax[1].set_ylim(
0,
)
fig.tight_layout()
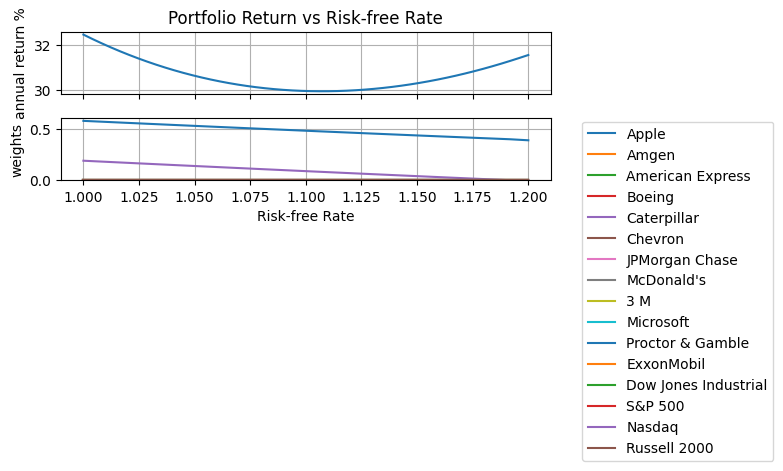
Extensions#
The examples cited in this notebook assume knowledge of the probability mass distribution. Recent work by Sun and Boyd (2018) and Hsieh (2022) suggest models for finding investment strategies for cases where the distributions are not perfectly known. They call them âdistributionally robust Kelly gambling.â A useful extension to this notebook would be to demonstrate a robust solution to one or more of the examples.
Bibliographic Notes#
Thorp, E. O. (2017). A man for all markets: From Las Vegas to wall street, how i beat the dealer and the market. Random House.
Thorp, E. O. (2008). The Kelly criterion in blackjack sports betting, and the stock market. In Handbook of asset and liability management (pp. 385-428). North-Holland. https://www.palmislandtraders.com/econ136/thorpe_kelly_crit.pdf
MacLean, L. C., Thorp, E. O., & Ziemba, W. T. (2010). Good and bad properties of the Kelly criterion. Risk, 20(2), 1. https://www.stat.berkeley.edu/~aldous/157/Papers/Good_Bad_Kelly.pdf
MacLean, L. C., Thorp, E. O., & Ziemba, W. T. (2011). The Kelly capital growth investment criterion: Theory and practice (Vol. 3). world scientific. https://www.worldscientific.com/worldscibooks/10.1142/7598#t=aboutBook
Carta, A., & Conversano, C. (2020). Practical Implementation of the Kelly Criterion: Optimal Growth Rate, Number of Trades, and Rebalancing Frequency for Equity Portfolios. Frontiers in Applied Mathematics and Statistics, 6, 577050. https://www.frontiersin.org/articles/10.3389/fams.2020.577050/full
The utility of conic optimization to solve problems involving log growth is more recent. Here are some representative papers.
Cajas, D. (2021). Kelly Portfolio Optimization: A Disciplined Convex Programming Framework. Available at SSRN 3833617. https://papers.ssrn.com/sol3/papers.cfm?abstract_id=3833617
Busseti, E., Ryu, E. K., & Boyd, S. (2016). Risk-constrained Kelly gambling. The Journal of Investing, 25(3), 118-134. https://arxiv.org/pdf/1603.06183.pdf
Fu, A., Narasimhan, B., & Boyd, S. (2017). CVXR: An R package for disciplined convex optimization. arXiv preprint arXiv:1711.07582. https://arxiv.org/abs/1711.07582
Sun, Q., & Boyd, S. (2018). Distributional robust Kelly gambling. arXiv preprint arXiv: 1812.10371. https://web.stanford.edu/~boyd/papers/pdf/robust_kelly.pdf
The recent work by CH Hsieh extends these concepts in important ways for real-world implementation.
Hsieh, C. H. (2022). On Solving Robust Log-Optimal Portfolio: A Supporting Hyperplane Approximation Approach. arXiv preprint arXiv:2202.03858. https://arxiv.org/pdf/2202.03858