Optimal Design of Multilayered Building Insulation#
Thermal insulation is installed in buildings to reduce annual energy costs. However, the installation costs money, so the decision of how much insulation to install is a trade-off between the annualized capital costs of insulation and the annual operating costs for heating and air conditioning. This notebook shows the formulation and solution of an optimization problem using conic optimization.
# install dependencies and select solver
%pip install -q amplpy numpy pandas
SOLVER_CONIC = "mosek" # ipopt, mosek, gurobi, knitro
from amplpy import AMPL, ampl_notebook
ampl = ampl_notebook(
modules=["coin", "mosek"], # modules to install
license_uuid="default", # license to use
) # instantiate AMPL object and register notebook magics
from IPython.display import Markdown, HTML
import numpy as np
import matplotlib.pyplot as plt
Please provide a valid license UUID. You can use a free https://ampl.com/ce license.
A Model for Multi-Layered Insulation#
Consider a wall or surface separating conditioned interior space in a building at temperature \(T_i\) from the external environment at temperature \(T_o\). Heat conduction through the wall is given by
where \(U\) is the overall heat transfer coefficient and and \(A\) is the heat transfer area. For a wall constructed from \(N\) layers of different insulating materials, the inverse of the overall heat transfer coefficient \(U\) is given by a sum of serial thermal âresistancesâ
where \(R_0\) is the thermal resistance of the structural elements. The thermal resistance of the \(n\)-th insulating layer is equal to \(R_n = \frac{x_n}{k_n}\) for a material with thickness \(x_n\) and a thermal conductivity \(k_n\), so we can rewrite
The economic objective is to minimize the cost \(C\), obtained as the combined annual energy operating expenses and capital cost of insulation.
We assume the annual energy costs are proportional to overall heat transfer coefficient \(U\) and let \(\alpha \geq 0\) be the coefficient for the proportional relationship of the overall heat transfer coefficient \(U\) to the annual energy costs. Furthermore, we assume the cost of installing a unit area of insulation in the \(n\)-th layer is given by the affine expression \(a_n + b_n x_n\). The combined annualized costs are then
where \(\beta\) is a discount factor for the equivalent annualized cost of insulation, and \(y_n\) is a binary variable that indicates whether or not layer \(n\) is included in the installation. The feasible values for \(x_n\) are subject to constraints
where \(T\) is an upper bound on insulation thickness.
Analytic solution for \(N=1\)#
In the case of a single layer, i.e., \(N=1\), we have a one-dimensional cost optimization problem of which we can obtain a close-form analytical solution directly. Indeed, the expression for the cost \(C(x)\) as a function of the thickness \(x\) reads
For fixed parameters \(k\), \(R_0\), \(\beta\), \(b\), we can calculate the optimum thickness \(x^*\) as
A plot illustrates the trade-off between energy operating costs and capital insulation costs and the corresponding optimal solution \(x^*\).
# application parameters
alpha = 30 # $ K / W annualized cost per sq meter per W/sq m/K
beta = 0.05 # equivalent annual cost factor
R0 = 2.0 # Watts/K/m**2
T = 0.30 # maximum insulation thickness
# material properties
k = 0.030 # thermal conductivity as installed
a = 5.0 # installation cost per square meter
b = 150.0 # installed material cost per cubic meter
import matplotlib.pyplot as plt
import numpy as np
f = lambda x: alpha / (R0 + x / k)
g = lambda x: beta * (a + b * x)
# solution
xopt = -k * R0 + np.sqrt(alpha * k / beta / b)
print(f"The optimal cost is equal to {f(xopt) + g(xopt):0.5f} per sq. meter")
print(f"The optimal thickness is {xopt:0.5f} meters\n")
# plotting
x = np.linspace(0, 1, 201)
fig, ax = plt.subplots(1, 1, figsize=(6.5, 4))
ax.plot(x, f(x), label="energy")
ax.plot(x, g(x), label="insulation")
ax.plot(x, f(x) + g(x), label="total", lw=3)
ax.plot(xopt, f(xopt) + g(xopt), ".", ms=10, label="minimum")
ax.legend()
ax.plot([0, xopt], [f(xopt) + g(xopt)] * 2, "r--")
ax.plot([xopt] * 2, [0, f(xopt) + g(xopt)], "r--")
ax.text(0, f(xopt) + g(xopt) + 0.3, f"{f(xopt) + g(xopt):0.3f}")
ax.text(xopt, 0.3, f"{xopt:0.3f}")
ax.set_xlabel("Insulation thickness [m]")
ax.set_ylabel("Cost ")
ax.set_title("Annualized costs of insulation and energy per sq. meter")
ax.grid(True)
The optimal cost is equal to 4.99615 per sq. meter
The optimal thickness is 0.28641 meters
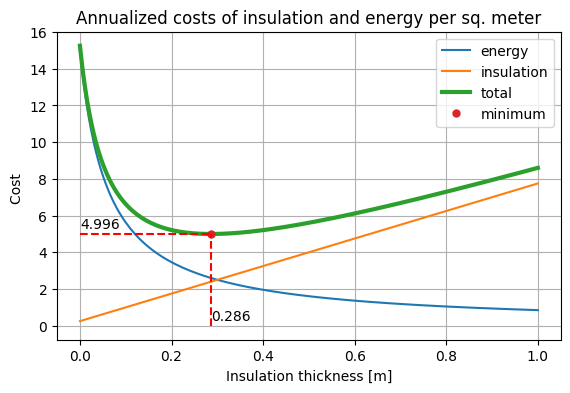
AMPL Model for \(N=1\)#
A conic rotated constraint in AMPL can be represented as follows:
where \(r_1, r_2\), and \(z_0, z_1, \ldots, z_{N-1}\) are variables. For a single layer AMPL model we identify \(R \sim r_1\), \(U\sim r_2\), and \(z_0^2 \sim 2\) which leads to the model
ampl = AMPL()
ampl.eval(
r"""
# application parameters
param alpha; # $ K / W annualized cost per sq meter per W/sq m/K
param beta; # equivalent annual cost factor
param R0; # Watts/K/m**2
param T; # maximum insulation thickness
# material properties
param k; # thermal conductivity as installed
param a; # installation cost per square meter
param b; # installed material cost per cubic meter
# decision variables
var R >= 0;
var U >= 0;
var x >= 0 <= T;
# objective
minimize cost: alpha*U + beta*(a + b*x);
# insulation model
s.t. r: R == R0 + x/k;
# conic constraint
s.t. q: R*U >= 1;
"""
)
ampl.param["alpha"] = alpha
ampl.param["beta"] = beta
ampl.param["R0"] = R0
ampl.param["T"] = T
ampl.param["k"] = k
ampl.param["a"] = a
ampl.param["b"] = b
ampl.option["solver"] = SOLVER_CONIC
ampl.solve()
print(
f"The optimal cost is equal to {ampl.get_objective('cost').value():0.5f} per sq. meter"
)
print(f"The optimal thickness is xopt = {ampl.get_value('x'):0.5f} meters")
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 4.996152429
0 simplex iterations
11 barrier iterations
The optimal cost is equal to 4.99615 per sq. meter
The optimal thickness is xopt = 0.28642 meters
Multi-Layer Solutions as a Mixed Integer Quadratic Constraint Optimization (MIQCO)#
For multiple layers, we cannot easily find an analytical optimal layer composition and we shall resort to conic optimization. Let \(y_n\) be the binary variable that indicates whether layer \(n\) is included in the insulation package or not, and \(x_n\) be the continuous variable describing the thickness of layer \(n\), which is zero if layer \(n\) is not included.
In the general case with \(N\) layers, the objective function is given by
where the first term is nonlinear in the variables \(x_1,\dots,x_N\) since since the denominator of the first term is equal tosince at the denominator of the first term is equal to
To overcome this issue, we can include \(U\) as a decision variable and include a constraint
Since we minimize the objective and \(U\) has no other constraint, the problem will guarantee that \(U\) is equal to \(1/R\). The extra constraint \(RU \leq 1\) can be reformulated using an extra decision variable \(z\) as:
from which we see that the entire problem can be reformulated as a conic optimization problem.
The middle formulation above can, in fact, be implemented in AMPL as a conic rotated constraint of the form:
In our case, we pick \(n=1\), \(z_0 = z\), \(r_1=R\), and \(r_2=U\).
Adopting this formulation, the full multi-layer optimization problem then reads:
def insulate(df, alpha, beta, R0, T):
ampl = AMPL()
ampl.eval(
r"""
# application parameters
param alpha; # $ K / W annualized cost per sq meter per W/sq m/K
param beta; # equivalent annual cost factor
param R0; # Watts/K/m**2
param T; # maximum insulation thickness
# material properties
set MAT;
param k{MAT}; # thermal conductivity as installed
param a{MAT}; # installation cost per square meter
param b{MAT}; # installed material cost per cubic meter
# decision variables
var R >= 0;
var U >= 0;
var x{MAT} >= 0;
var y{MAT} binary;
# objective
minimize cost:
alpha*U + beta * sum {i in MAT} (a[i] + b[i]*x[i]);
# insulation model
s.t. insulation: R == R0 + sum {i in MAT} x[i]/k[i];
# total thickness limit
s.t. thickness: sum {i in MAT} x[i] <= T;
# layer model
s.t. layers {i in MAT}:
x[i] <= T * y[i];
# conic constraint
s.t. q: R*U >= 1;
"""
)
ampl.param["alpha"] = alpha
ampl.param["beta"] = beta
ampl.param["R0"] = R0
ampl.param["T"] = T
ampl.set["MAT"] = list(df.index)
ampl.set_data(df, "MAT")
ampl.option["solver"] = SOLVER_CONIC
ampl.solve()
df["x_opt"] = ampl.get_variable("x").to_pandas()
print(
f"\nThe optimal cost is equal to"
f" {ampl.get_objective('cost').value():0.5f} per sq. meter"
)
display(df.round(5))
return ampl
Case 1. Single Layer Solution#
import pandas as pd
# application parameters
alpha = 30 # $ K / W annualized cost per sq meter per W/sq m/K
beta = 0.05 # equivalent annual cost factor
R0 = 2.0 # Watts/K/m**2
T = 0.30 # maximum insulation thickness
df = pd.DataFrame(
{
"Mineral Wool": {"k": 0.030, "a": 5.0, "b": 150.0},
"Rigid Foam (high R)": {"k": 0.015, "a": 8.0, "b": 180.0},
"Rigid Foam (low R)": {"k": 0.3, "a": 8.0, "b": 120.0},
}
).T
m = insulate(df, alpha, beta, R0, T)
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 4.804922361
0 simplex iterations
1 branching nodes
The optimal cost is equal to 4.80492 per sq. meter
k | a | b | x_opt | |
---|---|---|---|---|
Mineral Wool | 0.030 | 5.0 | 150.0 | 0.00000 |
Rigid Foam (high R) | 0.015 | 8.0 | 180.0 | 0.19361 |
Rigid Foam (low R) | 0.300 | 8.0 | 120.0 | 0.00000 |
Case 2. Multiple Layer Solution#
# application parameters
alpha = 30 # $ K / W annualized cost per sq meter per W/sq m/K
beta = 0.05 # equivalent annual cost factor
R0 = 2.0 # Watts/K/m**2
T = 0.15 # maximum insulation thickness
df = pd.DataFrame(
{
"Foam": {"k": 0.015, "a": 0.0, "b": 110.0},
"Wool": {"k": 0.010, "a": 0.0, "b": 200.0},
}
).T
m = insulate(df, alpha, beta, R0, T)
MOSEK 10.0.43: MOSEK 10.0.43: optimal; objective 3.229922362
0 simplex iterations
1 branching nodes
The optimal cost is equal to 3.22992 per sq. meter
k | a | b | x_opt | |
---|---|---|---|---|
Foam | 0.015 | 0.0 | 110.0 | 0.06278 |
Wool | 0.010 | 0.0 | 200.0 | 0.08722 |
The plot below gives a graphical representation of the 2-layer problem we just solved. The green line represents the thickness constraint \(x_0+x_1 \leq T\), the curves are the isolines of the objective function, and the optimal solution \(x^*=(x_0^*,x_1^*)\) is highlighted in red.
import numpy as np
import matplotlib.pyplot as plt
k = list(df["k"])
a = list(df["a"])
b = list(df["b"])
f = lambda x0, x1: alpha / (R0 + x0 / k[0] + x1 / k[1]) + beta * (
a[0] + b[0] * x0 + a[1] + b[1] * x1
)
x0 = np.linspace(0, 1.1 * T, 201)
x1 = np.linspace(0, 1.1 * T, 201)
X0, X1 = np.meshgrid(x0, x1)
fig, ax = plt.subplots(1, 1)
ax.contour(x0, x1, f(X0, X1), 50)
ax.set_xlim(min(x0), max(x0))
ax.set_ylim(min(x1), max(x1))
ax.plot([0, T], [T, 0], "g", lw=2.5)
ax.set_aspect(1)
x = df["x_opt"].to_list()
ax.plot(x[0], x[1], "r.", ms=20)
ax.text(x[0], x[1], f" ({x[0]:0.4f}, {x[1]:0.4f})")
ax.set_xlabel(r"$x_0$")
ax.set_ylabel(r"$x_1$")
ax.set_title("Contours of Constant Cost")
plt.show()
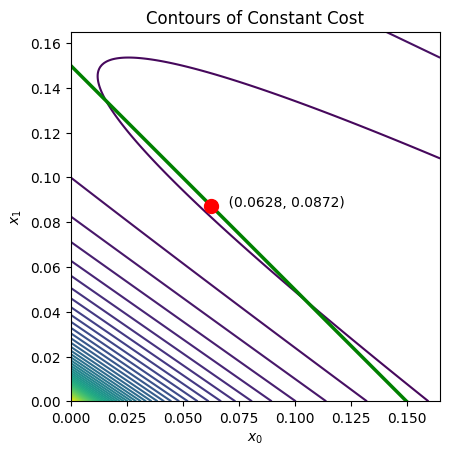
Bibliographic Notes#
To the best of my knowledge, this problem is not well-known example in the mathematical optimization literature. There are a number of application papers with differing levels of detail.
Hasan, A. (1999). Optimizing insulation thickness for buildings using life cycle cost. Applied energy, 63(2), 115-124. https://www.sciencedirect.com/science/article/pii/S0306261999000239
Kaynakli, O. (2012). A review of the economical and optimum thermal insulation thickness for building applications. Renewable and Sustainable Energy Reviews, 16(1), 415-425. https://www.sciencedirect.com/science/article/pii/S1364032111004163
Nyers, J., Kajtar, L., TomiÄ, S., & Nyers, A. (2015). Investment-savings method for energy-economic optimization of external wall thermal insulation thickness. Energy and Buildings, 86, 268-274. https://www.sciencedirect.com/science/article/pii/S0378778814008688
More recently some modeling papers have appeared
Gori, P., Guattari, C., Evangelisti, L., & Asdrubali, F. (2016). Design criteria for improving insulation effectiveness of multilayer walls. International Journal of Heat and Mass Transfer, 103, 349-359. https://www.sciencedirect.com/science/article/abs/pii/S0017931016303647
Huang, H., Zhou, Y., Huang, R., Wu, H., Sun, Y., Huang, G., & Xu, T. (2020). Optimum insulation thicknesses and energy conservation of building thermal insulation materials in Chinese zone of humid subtropical climate. Sustainable Cities and Society, 52, 101840. https://www.sciencedirect.com/science/article/pii/S221067071931457X
Söylemez, M. S., & Ănsal, M. (1999). Optimum insulation thickness for refrigeration applications. Energy Conversion and Management, 40(1), 13-21. https://www.sciencedirect.com/science/article/pii/S0196890498001253
Açıkkalp, E., & Kandemir, S. Y. (2019). A method for determining optimum insulation thickness: Combined economic and environmental method. Thermal Science and Engineering Progress, 11, 249-253. https://www.sciencedirect.com/science/article/pii/S2451904918305377
Ylmén, P., Mjörnell, K., Berlin, J., & Arfvidsson, J. (2021). Approach to manage parameter and choice uncertainty in life cycle optimisation of building design: Case study of optimal insulation thickness. Building and Environment, 191, 107544. https://www.sciencedirect.com/science/article/pii/S0360132320309112